Software development is the field to which our blog is devoted to. Therefore, we could not miss an article discussing one of the most important issues: the best practices for software development.
Of course, this is such a broad field that it is impossible to list all aspects, but there are a few most important ones that, if properly implemented in the software development process, can make the life of the software development team significantly easier.
Without further ado, let’s take a look at the best software engineering practices.
YAGNI
This acronym stands for “You Ain’t Gonna Need It.” In software development, this principle states that a software developer should not add functionality to a system that is not currently needed.
Adding unneeded functionality increases complexity and can lead to code bloat. It can also introduce bugs and security vulnerabilities. In some cases, it may be impossible to remove unneeded functionality once it has been added.
YAGNI is often used in conjunction with the principle of KISS (Keep It Simple, Stupid). Together, these principles help developers create systems that are easy to understand, maintain, and extend.
YAGNI is also sometimes misinterpreted as meaning that all code should be written as if it will never need to be changed. This can lead to inflexible code that is difficult to maintain. YAGNI should be applied to strike a balance between flexibility and simplicity.
Moreover, software projects are often produced over a long period, resulting in minor or major technological changes. Therefore, there is no need to waste time creating unnecessary modules that may turn out to be useless later. Instead, it’s better to fine-tune the functionalities that are needed here and now.
KISS
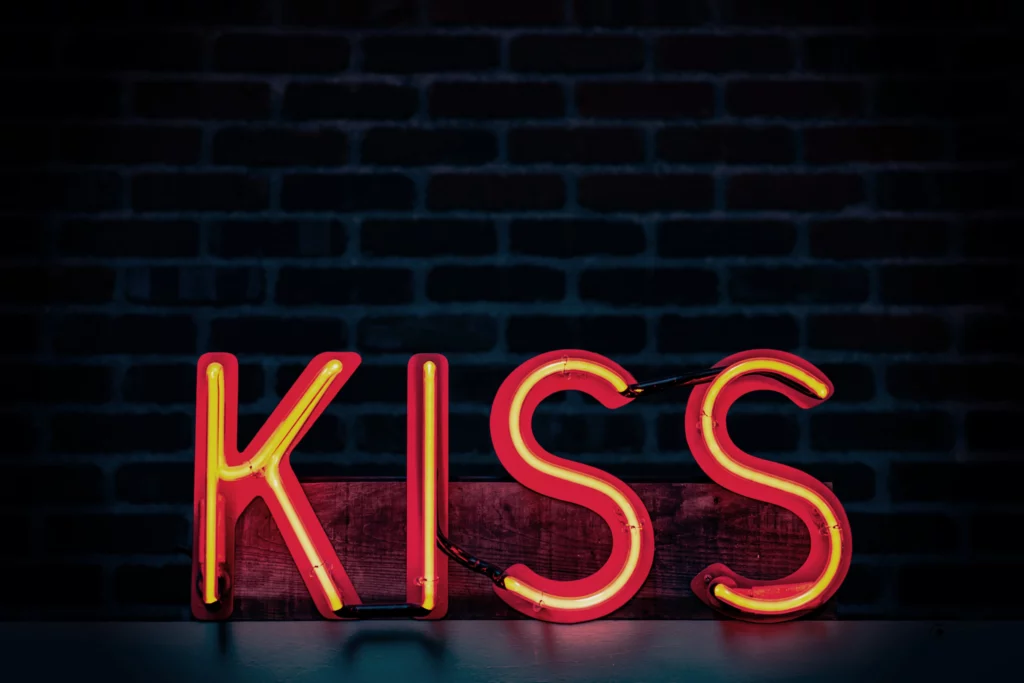
We mentioned this method as a great complement to YAGNI. As the name (Keep It Simple Stupid) suggests, the idea is to keep things as simple as possible. This will make your code more readable and easier to maintain in the long run. Cutting down on unnecessary coding is probably the best you can do when creating software because the less code, the less chance of errors. The KISS principle is often associated with the Unix philosophy – „do one thing and do it well.” In one word – keep your code simple.
DRY
Another great principle to follow is DRY or Don’t Repeat Yourself. This means that you should avoid writing duplicate code as much as possible. Duplicate code is not only harder to read and maintain, but it’s also more error-prone. If you need to change to duplicated code, you must remember to make the same change in all places where that code appears. It’s much better to write a single piece of code and reuse it wherever necessary.
Code refactoring
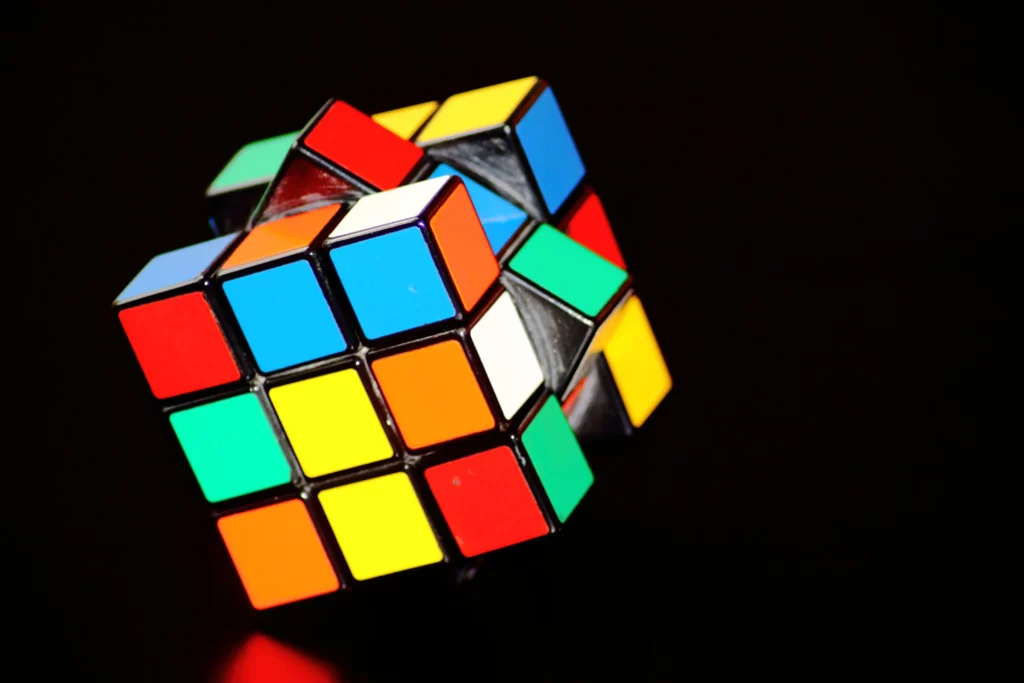
Refactoring is the process of improving the design of existing code without changing its functionality. It’s a great way to improve the code quality and make it more maintainable. When you refactor code, you restructure it without changing its behavior. This can be done by extracting methods, renaming variables, or moving classes to other files.
There are many different techniques you can use to refactor code. The most important thing is to do it regularly. As your codebase grows, it will become more difficult to make large-scale changes. That’s why it’s important to refactor often to keep your code clean and manageable.
Unit testing
Unit testing is automated testing where each software program component is tested to see if it works properly. In unit testing, small pieces of code, called units, are tested in isolation from the rest of the program. This helps to ensure that each unit works correctly before the entire program is put together.
Unit testing is an important part of the software development process. It helps to find bugs early on and prevents them from becoming bigger issues later. Unit tests should be written before any code is written. That way, you can be sure that your code does what it’s supposed to do.
Integration testing
Integration testing is automated testing where different software program components are tested to see if they work together correctly. This method differs from unit testing, where individual units are tested in isolation.
Integration tests are important because they help to find bugs that can only be found when two or more components are used together. They also help to ensure that the different components of a software program work together correctly.
Continuous testing
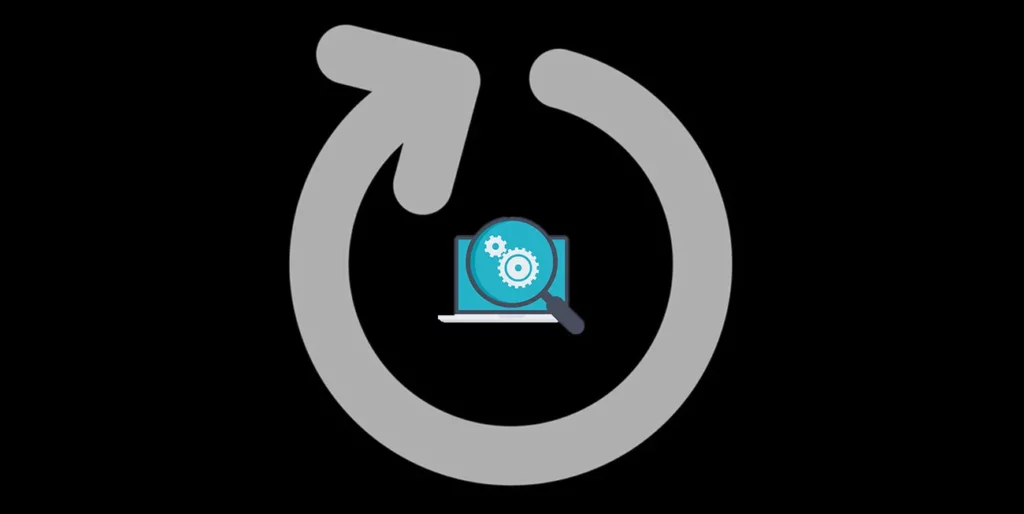
Both integration testing and unit testing should be performed on an ongoing basis, regardless of the software development lifecycle stage, you are on. Don’t wait until your code is fully built, as it may turn out to be too late to test and have so many bugs that it will be hard to fix. Continuous testing will also allow you to understand better what has already been created and show you which areas still need to be refined.
One of the benefits of continuous testing is that it can help to avoid “integration hell.” This is when a software program has so many dependencies and moving parts that it becomes difficult or impossible to test. By testing early and often, you can avoid this problem.
Code reviews
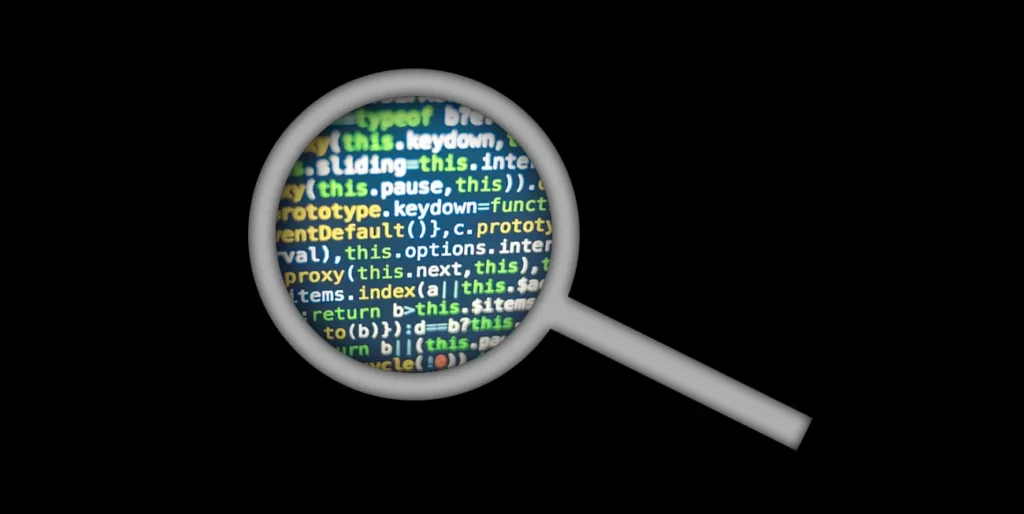
Code reviews are a way to get feedback on your code from other developers. Code reviews can be done in a number of ways. One popular method is to have one or more people review the code and then provide feedback. Another way is to use a tool that automatically checks the code for errors.
In general, it is best to have some kind of code review process in place. This will help to ensure that code meets software development standards and is of high quality.
Reviewing the source code can also lower the chances of technical debt, as old and redundant pieces of code can be found and erased. Remember that at least one person should not be the code creator when doing code reviews.
Documentation
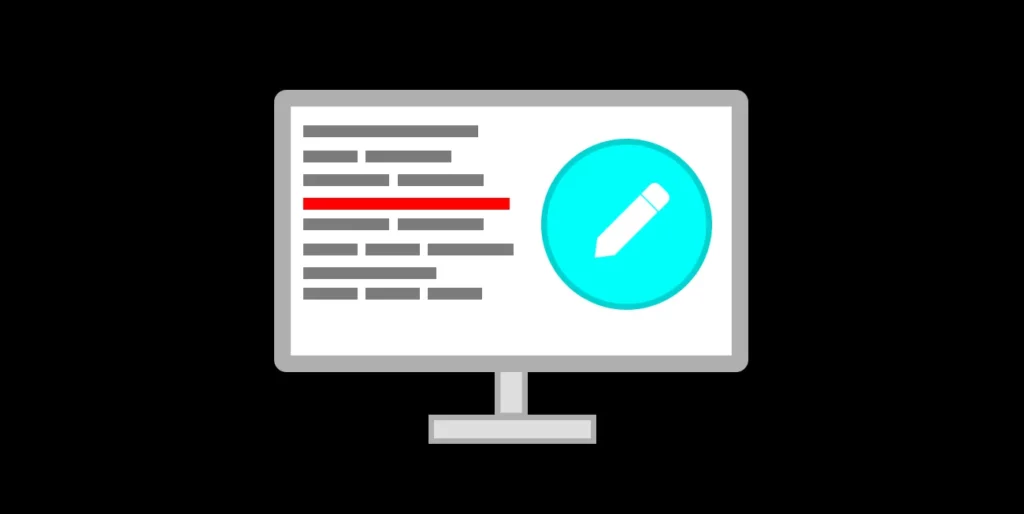
Documentation is important for software development projects. It can help to ensure that everyone on the team understands the code and how it works. Good documentation can also be helpful for future developers who may need to work on the code.
There are many different ways to document code. One popular method is to use comments within the code itself. Another way is to create separate documentation files that describe the code. When creating documentation, be sure to include information on how to install and run the code, as well as an overview of its features.
It is also important to keep documentation up to date. As code changes, updated documentation can help everyone on the team understand the new changes.
Estimation
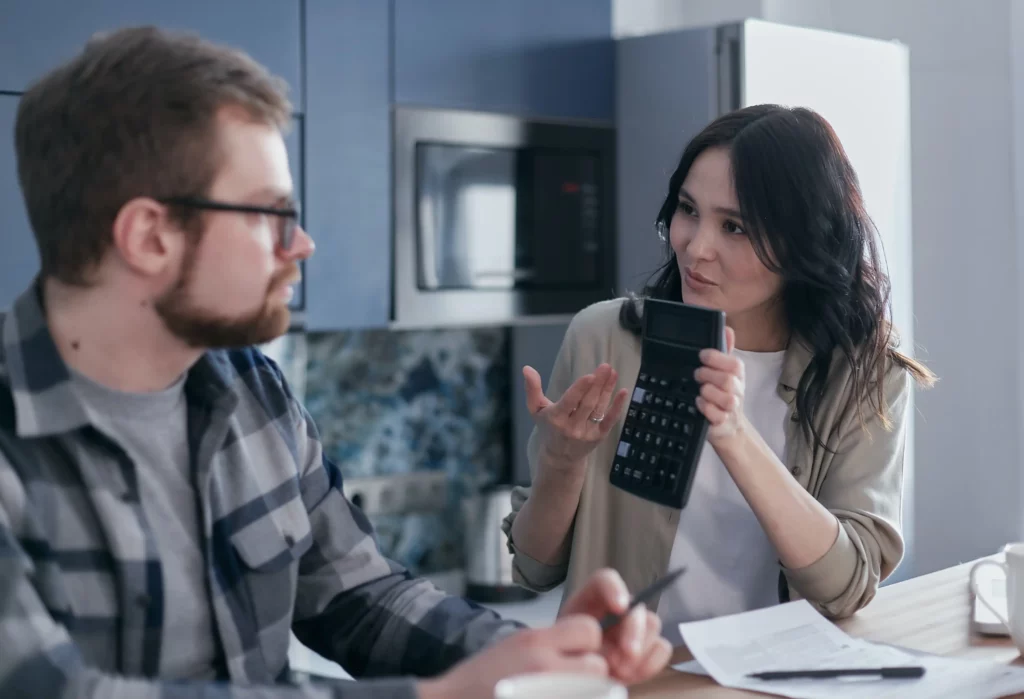
Estimation is one of the most difficult aspects of a software project, and it is often where the project goes wrong. Estimating a project’s time, resources, and cost can be a complex task, and there are many factors to consider.
When estimating a project, it is important to be as realistic as possible. Have all the stakeholders involved in the estimation process, and ensure everyone understands the project’s scope. It is also important to break down the estimate into smaller pieces to make it easier to track progress and adjust as needed.
Common mistake
One common mistake when estimating projects is underestimating the time and effort required. This can lead to problems later on in the project when deadlines are not met, or budget overruns occur.
Estimation is not exactly a step that software developers should carry out, but that should be a part of it because they know best how much time and effort it will take to make the app running.
Use frameworks / SDKs
Frameworks, and SDKs, are great tools that can significantly speed up the development process and lower costs.
They provide a set of well-tested, reusable components that can be integrated into your project with minimal effort. This can save you a lot of time and money that would otherwise be spent on developing similar functionality from scratch.
Whenever possible, use existing frameworks and SDKs to speed up development and reduce costs.
The most popular frameworks/SDKs for mobile development
- React Native
- Flutter
- Ionic
- Swift
- Kotlin
- Learn more in our article
The most popular frameworks/SDKs for web development
- Laravel
- React JS
- Node JS
- Symfony
- Angular
- .NET
Do not reinvent the wheel
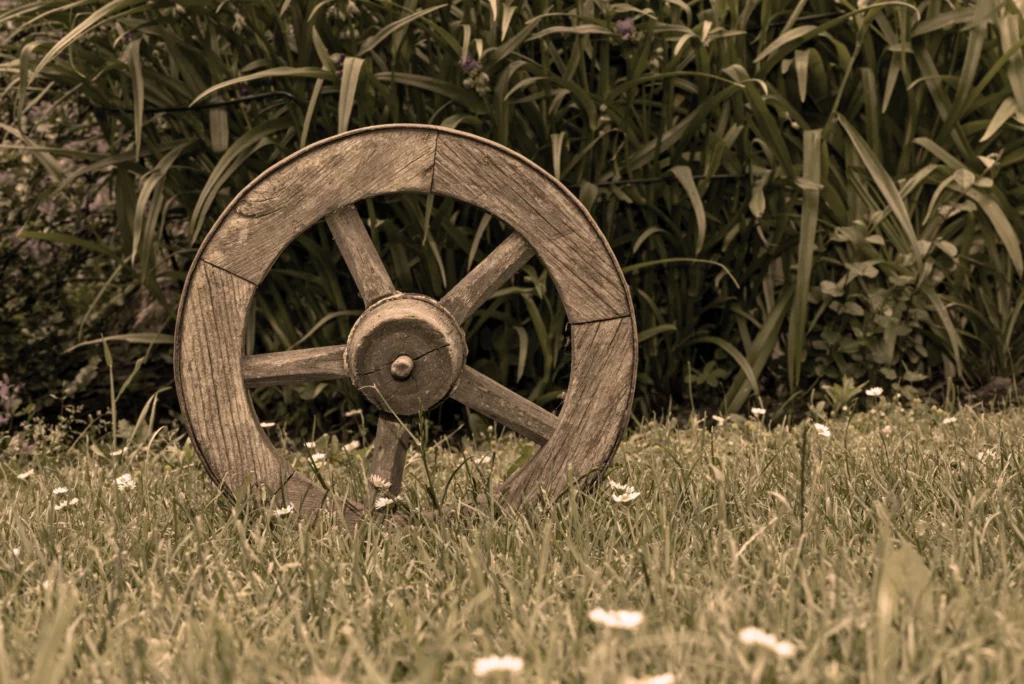
It is generally better to use existing solutions rather than trying to develop everything from scratch. Not only will this save you time and money, but it will also ensure that your project benefits from the collective experience of the developer community.
There are many great open source projects available that can be used in your own projects. By using existing solutions, you can avoid making common mistakes that have already been solved by others.
In addition, existing solutions are often more robust and well-tested than something you could develop on your own. When using third-party components, be sure to vet them carefully to ensure that they meet your quality standards.
Use version control
Version control is a critical tool for any software development project. It allows you to track changes to your code over time and makes it easy to revert back to previous versions if necessary.
Many different version control systems are available, but Git is one of the most popular. GitHub is a popular hosting service for Git repositories that also offers many features for collaboration and code review.
Before starting your project, familiarize yourself with the basics of version control.
Plan for change
No matter how well you plan, your software development project will likely change over time. You should anticipate this by planning for change from the start.
Use a flexible development methodology like Agile or Lean that encourages constant adaptation. Make sure your project management tool can handle changes easily and use features like kanban boards to help you visualize and track progress.
Be prepared to make changes to your code as well. Use techniques like refactoring to keep your code clean and easy to modify. And always write tests! Automated tests will save you a lot of time and effort in the long run by catching bugs early on.
Conclusion
As we stated at the very beginning of this article, software development is a vast topic that we could write about day and night. That is why we picked only a few of the best software development practices that we believe can impact your project the most. We hope that the knowledge related to developing software collected in this article will allow you to develop a successful web application or mobile application without any problems along the way.